Design systems have become increasingly important for web development in recent years. A react design system is a collection of reusable react components, guidelines, and assets that help to maintain consistency and improve the user experience across a product or application.
React and Typescript are two technologies that are well-suited for building design systems. React provides a component-based architecture that makes it easy to create reusable UI components, while Typescript adds static typing and other features that help to improve code quality and maintainability.
In this blog post, we’ll show you how to set up your own react native design system with React and Typescript. We’ll walk you through the process of planning your design system, setting up your React + Typescript project, creating your component library, styling your components, and documenting your design system.
What is a Design System?
Before getting started, it’s important to define what is a design system. A design system is a collection of reusable UI components, guidelines, and assets that help to maintain consistency and improve the user experience across a product or application.
A design system typically includes the following components:
- Design principles: A set of guiding principles that define the overall look and feel of the product or application.
- Style guide: A set of guidelines that define the typography, color palette, and other design elements that are used throughout the product or application.
- Component library: A collection of reusable UI components that can be used to build the product or application.
- Documentation: A set of documentation that describes how to use the design system and its components.
React and Typescript for Design Systems?
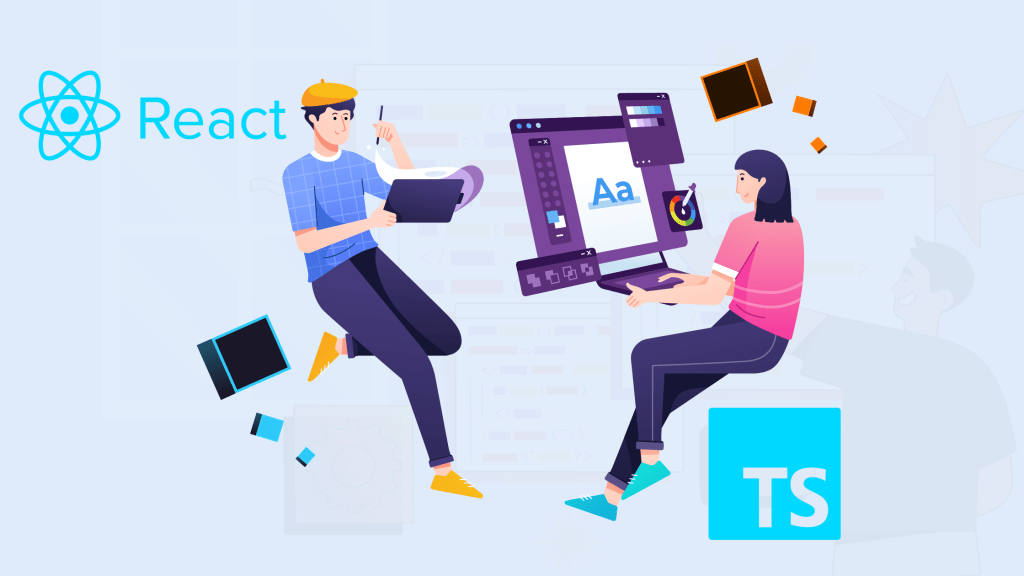
React is a popular JavaScript library for building user interfaces. It provides a component-based architecture that makes it easy to create reusable UI components. React also includes features like virtual DOM and JSX that help to improve performance and reduce development time.
Typescript is a superset of JavaScript that adds static typing and other features to the language. Typescript can help to improve code quality and maintainability by catching errors at compile-time rather than run-time.
When used together, React and Typescript can help to improve the quality and maintainability of your react native design system. React provides a component-based architecture for building reusable UI components, while Typescript adds static typing and other features that help to catch errors and improve code quality.
Planning Your Design System
Before you start building your react design system, it’s important to plan it out. Here are a few things to consider:
- Define your design principles: Think about the overall look and feel that you want for your product or application. What design principles will guide the development of your design system?
- Create a style guide: Define the typography, color palette, and other design elements that will be used throughout your product or application. Create a style guide that documents these elements.
- Establish a component library: Think about the UI components that you’ll need to build your product or application. Which components can be reused across the application? Establish a component library that includes these components.
- Define your documentation strategy: Consider how you’ll document your react design system and its components. Will you use a living style guide like Storybook, or will you create a separate documentation site?
Setting Up Your React + Typescript Project
Now that you’ve planned out your react native design system, it’s time to set up your React + Typescript project. Here are the steps to follow:
- Create a new React app using create-react-app:
npx create-react-app my-app --template typescript
- Install additional packages:
npm install styled-components @types/styled-components
npm install styled-system @types/styled-system
These commands create your project folder, which looks like this:
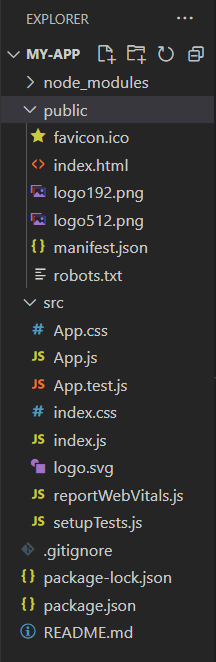
Creating Your Component Library
Now that your React + Typescript project is set up, it’s time to start building your component library. Here are the steps to follow:
- Create a new folder in your project called “components“.
- Inside the “components” folder, create a new folder for each component that you’ll be building. For example, you might create folders for “Button“, “Input“, and “Dropdown“.
- Inside each component folder, create a new file called “index.tsx“. This file will contain the component code.
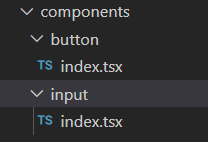
- Start by creating a basic functional component using the React.FC interface. Here’s an example for a Button component:
import React from 'react';
interface ButtonProps {
children: React.ReactNode;
}
const Button: React.FC<ButtonProps> = ({ children }) => {
return <button>{children}</button>;
};
export default Button;
- Next, add props to your component as needed. For example, you might add a “variant” prop to your Button component to change its appearance:
import React from 'react';
import styled from 'styled-components';
interface ButtonProps {
children: React.ReactNode;
variant?: 'primary' | 'secondary';
}
const Button: React.FC<ButtonProps> = ({ children, variant = 'primary' }) => {
return <StyledButton variant={variant}>{children}</StyledButton>;
};
const StyledButton = styled.button<ButtonProps>`
background-color: ${(props) =>
props.variant === 'primary' ? 'blue' : 'gray'};
color: white;
padding: 8px 16px;
border-radius: 4px;
border: none;
`;
export default Button;
- Finally, export your component from the “index.tsx” file. For example:
export { default } from './Button';
Styling Your Components
Once you’ve created your component library, it’s time to style your components. There are many ways to style React components, but one popular approach is to use a library like styled-components or emotion.
In our example, we’ll use styled-components to style our components. Here’s an example for styling our Button component:
import styled from 'styled-components';
interface ButtonProps {
children: React.ReactNode;
variant?: 'primary' | 'secondary';
}
const Button = styled.button<ButtonProps>`
background-color: ${(props) =>
props.variant === 'primary' ? 'blue' : 'gray'};
color: white;
padding: 8px 16px;
border-radius: 4px;
border: none;
`;
export default Button;
With styled-components, we can define our component styles using a template literal syntax. We can also pass props to our styled components, as shown in the example above.
Documenting Your Design System
Finally, it’s important to document your design system and its components. There are many tools and frameworks available for documenting React components, but one popular option is Storybook.
Storybook is a tool for building UI component libraries and documenting them with live examples. With Storybook, you can create a living style guide that shows your components in action.
To set up Storybook for your design system, follow these steps:
- Install the @storybook/cli package:
npm install -g @storybook/cli
- Run the following command to set up Storybook in your project:
sb init
- This will create a new “storybook” folder in your project. Inside this folder, you can create stories for your components. A story is a live example of a component in action.
- Here’s an example of a story for our Button component:
import React from 'react';
import { Story, Meta } from '@storybook/react';
import Button from '../components/Button';
export default {
title: 'Button',
component: Button,
} as Meta;
const Template: Story = (args) => <Button {...args} />;
export const Primary = Template.bind({});
Primary.args = {
variant: 'primary',
children: 'Primary Button',
};
export const Secondary = Template.bind({});
Secondary.args = {
variant: 'secondary',
children: 'Secondary Button',
};
In this example, we define a story for our Button component with two different variants: “Primary” and “Secondary”. We use the Storybook Template
component to render our Button
component with the args
passed in. Finally, we export our Primary
and Secondary
stories using the Template
component.
With this set up, you can now view your component library in Storybook and see how your components look and behave in different scenarios. This can be a great way to ensure consistency and usability across your design system.
Conclusion
Setting up your own design system with React + Typescript can be a challenging task, but it’s well worth the effort. By creating a component library, styling your components, and documenting your design system, you can build a reusable and scalable system for your web applications.
Ishan (Frontend developer at Fountane) describes one more way to build a design system using only React and TypeScript: here.
Whether you’re building a small project or a large enterprise application, a design system can help you save time, reduce costs, and improve the user experience. So why not give it a try and see how it can benefit your team and your users?
Leave feedback about this